在這個例子MVC的架構中,希望能夠套用jQuery .Ajax()的方式,透過透過POST方式呼叫給Controller中的Action,經過Action處理後(可能是查詢或存取後端資料庫處理元件),透過jQuery UI progress bar的介面方式,回應目前系統處理狀態在使用者所瀏覽的網頁上。
首先使用VS Web Developer 2010 Express建立一個ASP.NET MVC的專案。
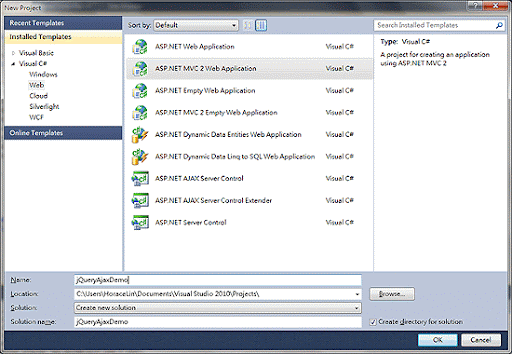
首先得引用jQuery,這裡我們使用Google API的方式,當然也可以將jQuery的相關js檔案直接加入我們的專案檔中來使用。
1: <script type="text/javascript" src="https://ajax.googleapis.com
/ajax/libs/jquery/1.4.3/jquery.min.js"></script>
2: <script type="text/javascript" src="https://ajax.googleapis.com
/ajax/libs/jqueryui/1.8.6/jquery-ui.min.js"></script>
接著,我們在HomeController中,加上兩個Action,可看到我們為了拉長Action處理時間,特別加上sleep()方法以更明顯來觀察progress bar的變化,並且回應一個「處理完成」的字串訊息如下:
1: public ActionResult jQueryDemo7()
2: {
3: return View();
4: }
5:
6: public ActionResult jQueryProgressBar()
7: {
8: for (int i = 0; i < 10; i++)
9: {
10: Thread.Sleep(100);
11: }
12: Response.Write("處理完成");
13: return null;
14: }
並且建立一個jQueryDemo7.aspx的View,然後加上jQuery的.ajax()語法,說明如下:
1.URL:這裡就是我們MVC架構中,我們要POST的/{Controller}/{Action},就是上面程式碼中的HomeController中的jQueryProgressBar這個Action。
2.type:我們使用POST的方式。
3.async:true表示我們需要使用同步即時的方式取得Server回應。
4.data:{},就是我們要這個範例並沒有傳給jQueryProgressBar這個Action任何參數。
5.datatype:"text",表示我們回傳回來的是text型態資料。
6.success:若執行完畢,則讓progress bar顯示長度為100%,並以html格式顯示「處理完成」的字串訊息至id=Results的<div>區塊內。
1.URL:這裡就是我們MVC架構中,我們要POST的/{Controller}/{Action},就是上面程式碼中的HomeController中的jQueryProgressBar這個Action。
2.type:我們使用POST的方式。
3.async:true表示我們需要使用同步即時的方式取得Server回應。
4.data:{},就是我們要這個範例並沒有傳給jQueryProgressBar這個Action任何參數。
5.datatype:"text",表示我們回傳回來的是text型態資料。
6.success:若執行完畢,則讓progress bar顯示長度為100%,並以html格式顯示「處理完成」的字串訊息至id=Results的<div>區塊內。
1: <h2>jQuery Ajax Mvc - jQuery UI Progress Bar</h2>
2: <p>使用jQuery UI 的prpgress bar效果</p>
3: <script type="text/javascript" language="javascript">
4: $(document).ready(function () {
5: $("#progressbar").progressbar({ value: 0 });
6: $("#btnSubmit").click(function () {
7: var intervalID = setInterval(updateProgress, 100);
8: $.ajax({
9: url: "/Home/jQueryProgressBar",
10: type: "POST",
11: async: true,
12: data: {},
13: dataType: "text",
14: success: function (data) {
15: $("#progressbar").progressbar("value", 100);
16: $("#Results").html(data);
17: clearInterval(intervalID);
18: }
19: });
20: return false;
21: });
22: });
23:
24: function updateProgress() {
25: var value = $("#progressbar").progressbar("option", "value");
26: if (value < 100) {
27: $("#progressbar").progressbar("value", value + 1);
28: }
29: }
30: </script>
31:
32: <div id="progressbar"></div>
33: <div id="Results"></div><br />
34: <input type="button" id="btnSubmit" value="Submit" />
我們就可以執行這個MVC專案,我們點選Demo7這個頁籤,如下圖:
然後按下Submit按鈕後,就看到隨著處理時間經過而顯示灰色bar由短變長的jQuery UI progress bar效果,如下圖:
系統處理完成後,我們收到Action傳來的「處理完成」的字串訊息,如下圖: